Jdk
查看java的相关信息 yum -y list java*
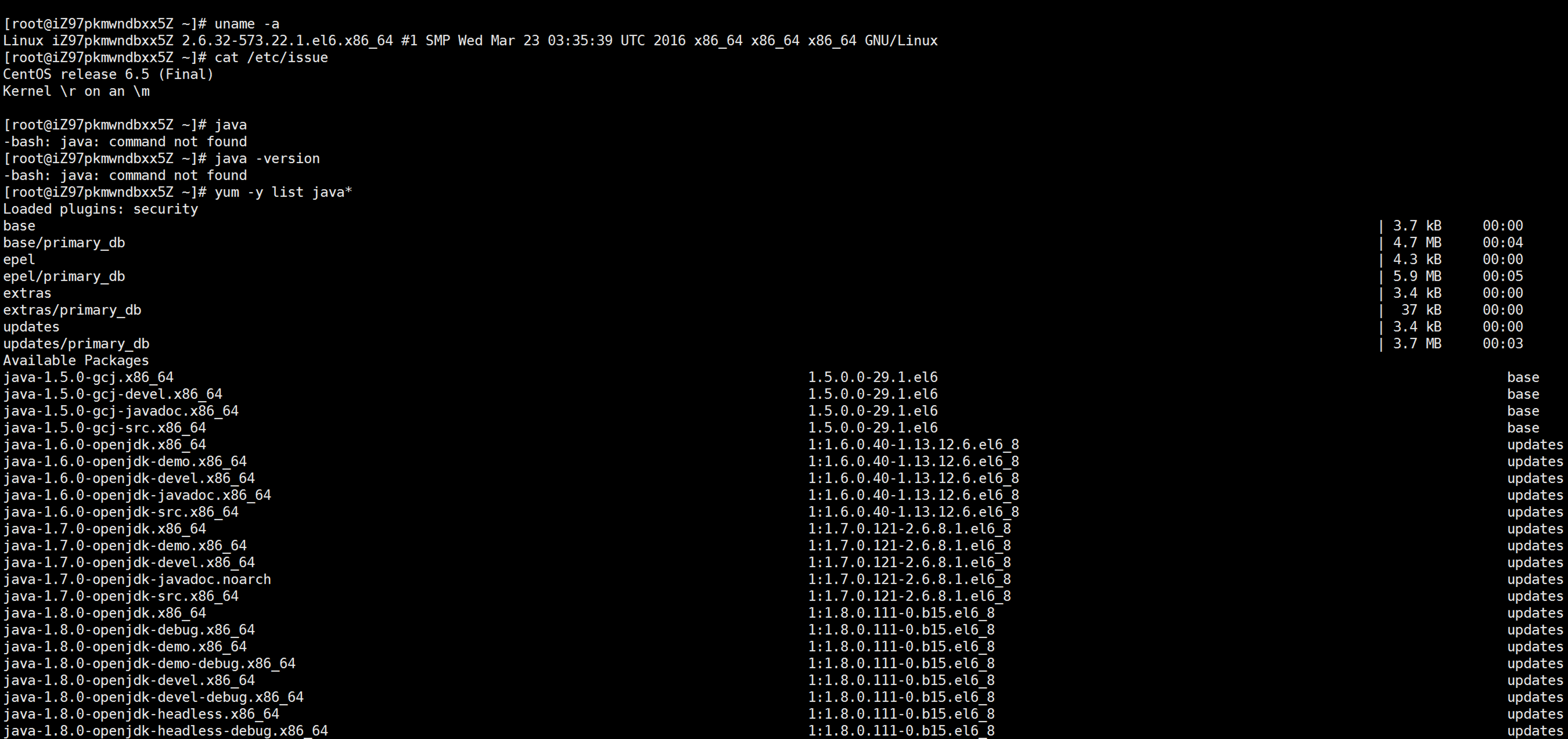
使用线程池可以有以下优点:
1 | /** |
1 | * This class provides thread-local variables. These variables differ from |
ThreadLocal
提供一种线程本地变量。这种变量是一种副本的概念,在多线程环境下访问(get、set)能够保证各个线程间的变量互相隔离。ThreadLocal
通常定义为了private static
,用来关联线程和线程上下文(比如userId或事物ID)。ThreadLocal
的作用是提供线程内的局部变量,这种变量在线程的生命周期内起作用,减少同一个线程内多个函数或者组件之间一些公共变量的传递的复杂度。